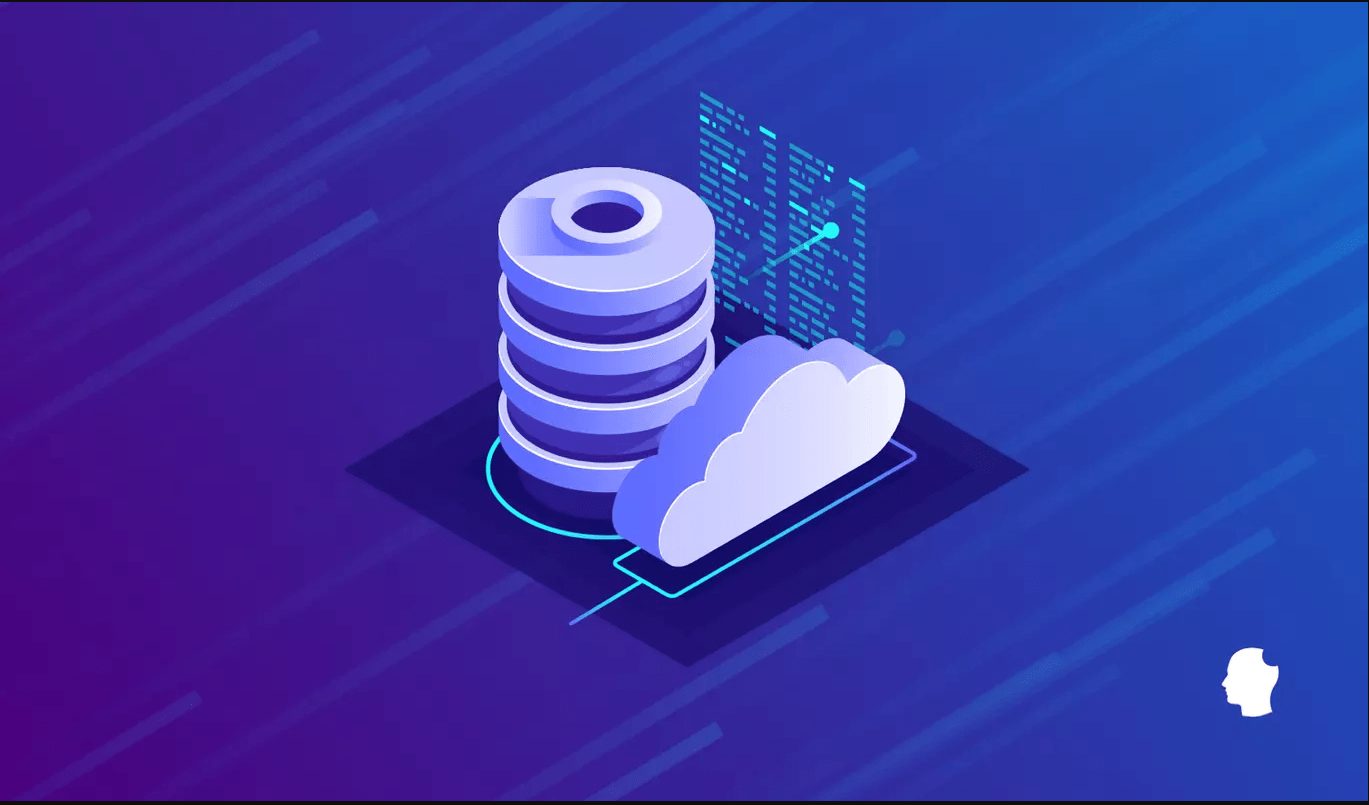
Query optimization is a critical aspect of database management, ensuring that your SQL queries run efficiently and your applications perform at their best. This guide delves into the nuances of query optimization, providing comprehensive insights and practical techniques to enhance your database performance.
What is Query Optimization?
Query optimization is the process of enhancing the performance of SQL queries to retrieve data from a database more efficiently. The goal is to minimize the resources required—such as CPU, memory, and disk I/O—while reducing the query execution time.
Why Query Optimization Matters
Efficient queries lead to faster application performance, reduced server load, and a better user experience. Poorly optimized queries can cause slow response times, increased server costs, and potential application failures.
Understanding the Query Execution Plan
A query execution plan is a roadmap that the database engine uses to execute a query. It shows how the database retrieves the requested data, detailing the steps and operations involved.
Viewing the Execution Plan
In SQL Server, you can view the execution plan using the EXPLAIN keyword:
EXPLAIN SELECT * FROM employees WHERE department = ‘Sales’;
For MySQL:
EXPLAIN SELECT * FROM employees WHERE department = ‘Sales’;
In PostgreSQL:
EXPLAIN ANALYZE SELECT * FROM employees WHERE department = ‘Sales’;
Key Elements of an Execution Plan
- Seq Scan: Sequentially scans all rows in the table.
- Index Scan: Uses an index to find rows more quickly.
- Nested Loop Join: Combines rows from two tables using a nested loop.
- Hash Join: Uses a hash table to join two tables.
Techniques for Query Optimization
1. Use Indexes Wisely
Indexes can significantly speed up data retrieval. However, they also introduce overhead for write operations. Use indexes on columns frequently used in WHERE, JOIN, and ORDER BY clauses.
Creating an Index
CREATE INDEX idx_department ON employees (department);
2. Avoid Select *
Selecting all columns can be inefficient, especially if you only need a few. Specify only the columns you need.
SELECT name, department FROM employees WHERE department = ‘Sales’;
3. Use Joins Efficiently
Joins can be expensive. Ensure that the joined columns are indexed and avoid unnecessary joins.
SELECT e.name, d.department_name
FROM employees eJOIN departments d ON e.department_id = d.id;
4. Optimize Subqueries
Subqueries can often be rewritten as joins or with EXISTS for better performance.
— Inefficient subquery
SELECT name FROM employees WHERE department_id IN (SELECT id FROM departments WHERE department_name = ‘Sales’);— Optimized with join
SELECT e.name
FROM employees e
JOIN departments d ON e.department_id = d.id
WHERE d.department_name = ‘Sales’;
5. Limit the Number of Rows Returned
Use LIMIT or TOP to restrict the number of rows returned by a query, especially in large datasets.
— MySQL
SELECT name FROM employees WHERE department = ‘Sales’ LIMIT 10;— SQL Server
SELECT TOP 10 name FROM employees WHERE department = ‘Sales’;
6. Analyze and Tune Slow Queries
Use profiling tools and query logs to identify and optimize slow queries. Tools like MySQL’s slow_query_log and SQL Server’s Query Store can be invaluable.
7. Partition Large Tables
Partitioning can help manage large tables by dividing them into smaller, more manageable pieces, improving query performance and maintenance.
External Resources
Conclusion
Query optimization is essential for maintaining high-performance database systems. By understanding execution plans, using indexes wisely, and applying various optimization techniques, you can ensure your queries run efficiently and your applications perform seamlessly.