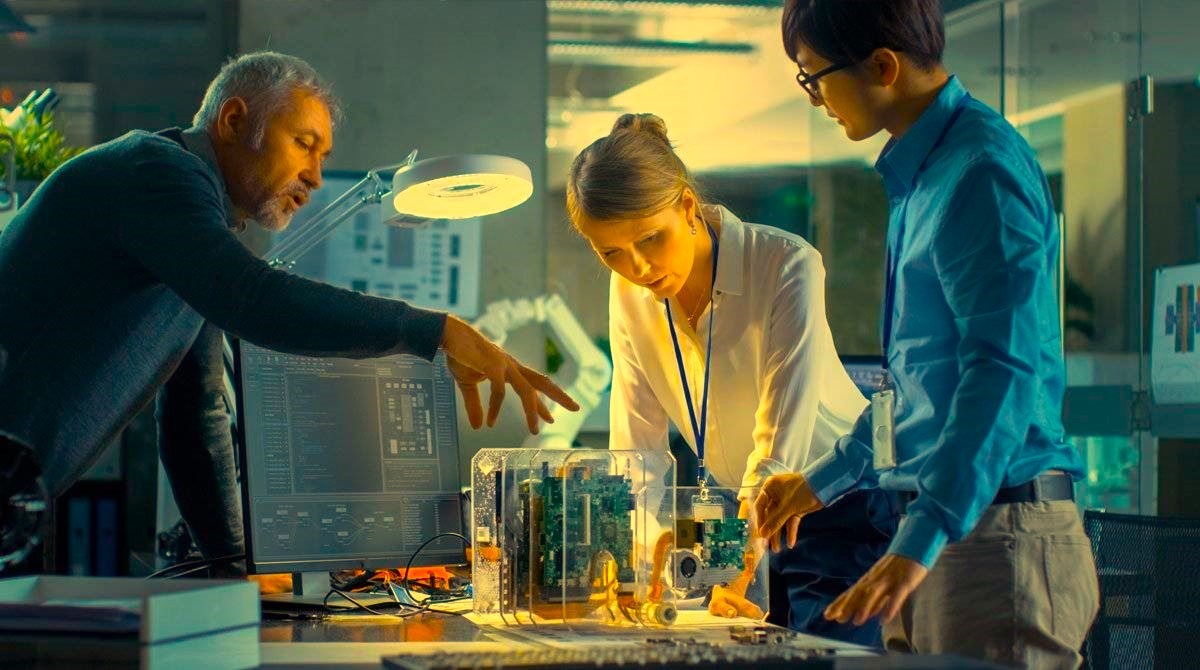
Understanding the Context Hook in ReactJS
ReactJS has revolutionized front-end development with its component-based architecture and powerful hooks. Among these hooks, the Context Hook stands out as a crucial tool for managing state and passing data through the component tree without prop drilling. In this blog, we will explore the Context Hook, its benefits, and how to use it effectively in your React applications.
What is the Context Hook?
The Context Hook, introduced in React 16.8, provides a way to share values like state or functions between components without passing props down manually at every level. It helps to avoid the “prop drilling” problem, where you have to pass props through several layers of components.
When to Use the Context Hook
The Context Hook is particularly useful in situations where data needs to be accessible by many components at different nesting levels. Common use cases include:
- Theme Management: Switching themes in an application.
- User Authentication: Sharing user information across the application.
- Localization: Providing language settings to various components.
For more detailed scenarios, you can refer to the official React documentation.
Creating a Context
Creating a context in React is straightforward. You need to:
- Create a Context object.
- Provide a value for the context.
- Consume the context value in the required components.
Here’s a simple example:
import React, { createContext, useContext, useState } from ‘react’;
// Create a Context
const ThemeContext = createContext();// Create a provider component
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState(‘light’);return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
};// Consume the context in a component
const ThemeSwitcher = () => {
const { theme, setTheme } = useContext(ThemeContext);return (
<div>
<p>Current Theme: {theme}</p>
<button onClick={() => setTheme(theme === ‘light’ ? ‘dark’ : ‘light’)}>
Toggle Theme
</button>
</div>
);
};const App = () => (
<ThemeProvider>
<ThemeSwitcher />
</ThemeProvider>
);export default App;
In this example, ThemeProvider
provides the theme context to its child components, and ThemeSwitcher
consumes it using the useContext
hook.
Benefits of the Context Hook
- Avoids Prop Drilling: Simplifies the passing of data through the component tree.
- Improves Code Maintainability: Makes the code cleaner and easier to manage.
- Encourages Reusability: Promotes reusability of components and context providers.
Performance Considerations
While the Context Hook is powerful, it should be used wisely. Overusing context can lead to performance issues, especially when frequent updates occur. To mitigate this, consider:
- Splitting context into smaller, more focused contexts.
- Using memoization techniques with hooks like
useMemo
anduseCallback
.
For in-depth performance tips, check out Kent C. Dodds’ article on optimizing context.
Conclusion
The Context Hook in ReactJS is an invaluable tool for managing state and sharing data across your application efficiently. By understanding and implementing the Context Hook properly, you can build more scalable and maintainable applications.
For further reading, visit React’s official guide on Context and explore more advanced patterns and techniques.
Understanding and leveraging the Context Hook can significantly enhance your React development workflow, making your applications more robust and easier to manage.